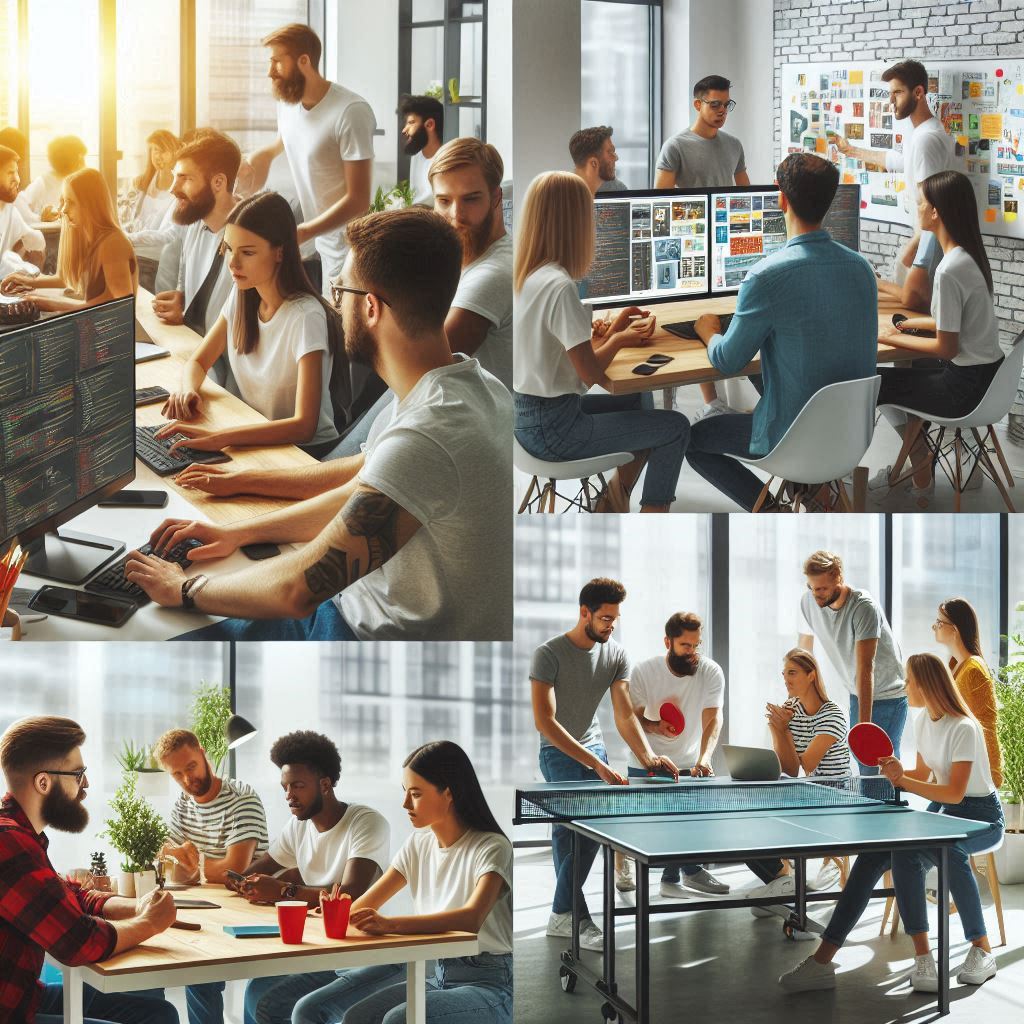
Looking to transform your ideas into immersive 3D experiences? ServReality offers cutting-edge Unity 3D development services designed to bring your project to life with the highest quality and technical expertise. Unity, as one of the most popular and versatile game engines, provides the flexibility and performance needed to create visually stunning, interactive applications, whether for games, simulations, or VR/AR experiences.
Why Choose ServReality for Unity 3D Development?
ServReality brings together a skilled team of developers, designers, and digital experts committed to delivering exceptional Unity-based solutions tailored to your specific goals. We understand the intricacies of the Unity engine and its capabilities in 2D, 3D, VR, and AR. Our team is equipped to handle everything from initial concept design to the final polished product, ensuring that your app or game exceeds expectations and keeps users engaged.
Our Unity 3D Development Services Include:
- Custom 3D Game Development: From concept to deployment, our team can create engaging games that run seamlessly across various platforms, including mobile, desktop, and consoles.
- Augmented and Virtual Reality Applications: With extensive experience in VR and AR, ServReality can create interactive applications that leverage Unity’s robust VR and AR capabilities.
- 3D Modeling and Animation: Our artists and developers produce lifelike 3D models and animations that enhance user experiences and add depth to your projects.
- Cross-Platform Optimization: Unity’s flexibility allows us to build apps for multiple platforms without compromising on quality, so your project can reach a broader audience.
Our Unity 3D development process at ServReality emphasizes close collaboration with our clients. This approach allows us to understand your vision and bring it to life in a way that captures the essence of your ideas. By utilizing Unity’s advanced tools and our own development experience, we ensure your project is visually impressive, performs efficiently, and stands out in a competitive market.
Unleash the Power of Unity for AR and VR
Unity is a preferred choice for AR and VR developers worldwide, thanks to its adaptability and robust set of features. From fully immersive VR simulations to interactive AR experiences, ServReality specializes in Unity-based AR and VR solutions that push the boundaries of what’s possible. If you’re interested in exploring more about Unity’s applications in AR and VR, check out this Wikipedia article for additional insights on Unity as a game engine.
Get Started with ServReality
When you choose ServReality, you’re partnering with a team dedicated to innovation, precision, and the pursuit of excellence in Unity 3D development. Our experience in game design, AR, VR, and 3D modeling ensures your project is in capable hands, ready to achieve its fullest potential. Connect with us today and take the first step towards realizing your Unity 3D vision with a team that is as passionate about your project as you are.
Ready to embark on your next project? Visit https://servreality.com/ to learn more about our Unity 3D services and how we can support your goals.