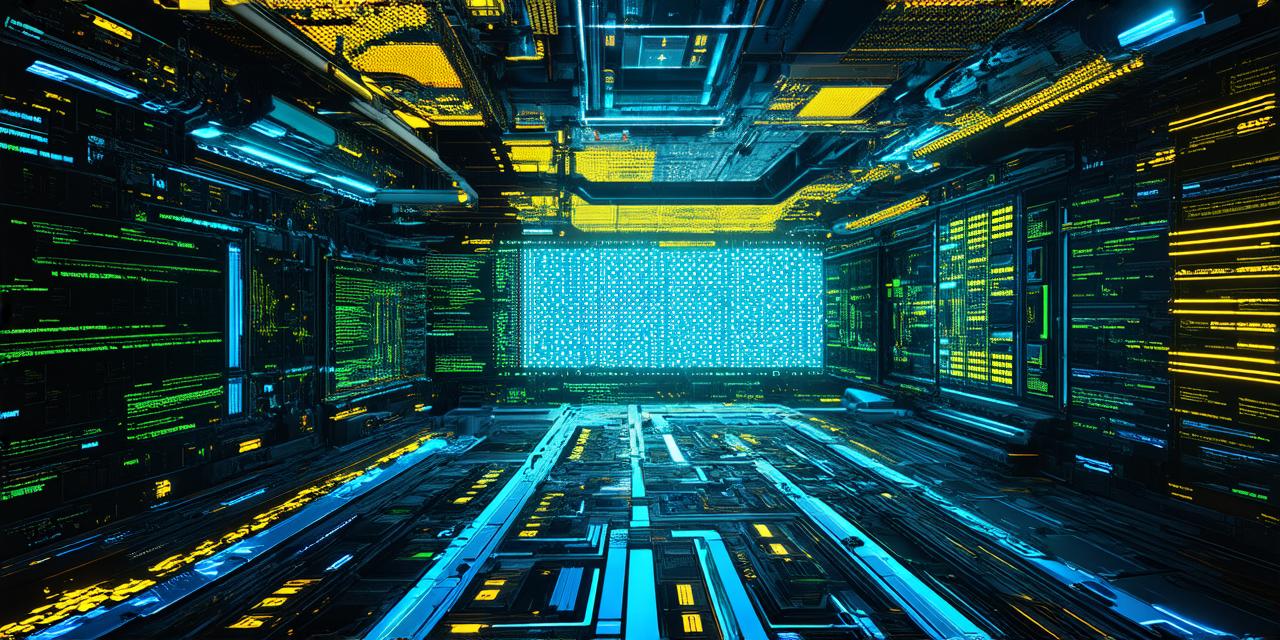
Introduction
Unity 3D is one of the most popular game engines on the market, with a massive community of developers who create everything from simple 2D games to complex virtual reality (VR) and augmented reality (AR) experiences. If you’re looking to learn how to program in Unity 3D, this comprehensive guide is the perfect place to start. In this article, we’ll cover everything from the basics of C scripting to advanced techniques for creating immersive VR and AR experiences.
Getting Started with Unity 3D and C Scripting
Before you can start programming in Unity 3D, you need to have a basic understanding of the software and its features. To get started, download and install the latest version of Unity from the official website (https://unity.com/). Once installed, create a new project and choose a template for your game or experience.
Once you have your project set up, it’s time to start writing code. Unity uses C as its primary scripting language, so if you’re already familiar with the language, you’ll be able to hit the ground running. If not, don’t worry – there are plenty of online resources and tutorials available that can help you get up to speed quickly.
One great way to learn C is by working through the official Unity Tutorials, which cover everything from basic scripting concepts like variables and functions to more advanced topics like networking and animation. You can access these tutorials directly within Unity or by visiting the Unity website (https://learn.unity.com/).
Creating Your First Game Object
Now that you have your project set up and are familiar with C scripting, it’s time to create your first game object. In Unity, a game object is any 3D asset that can be interacted with in the scene, such as characters, vehicles, or environmental elements like buildings or trees.
To create a new game object, select the "GameObject" menu at the top of the Unity editor and choose the type of object you want to create (e.g., sphere, cube, plane). Once you’ve created your object, you can customize its appearance and behavior using scripting.
For example, let’s say you’ve created a new sphere game object. To make it move around the scene, you could write a simple C script that sets its velocity and direction:
csharp
using UnityEngine;
public class SphereMovement : MonoBehaviour
{
public float speed = 5f; // how fast the sphere moves
public Vector3 direction = Vector3.forward; // where the sphere is moving
void Update()
{
transform.position += speed direction Time.deltaTime;
}
}
This script sets the sphere’s velocity (speed) and direction, and then updates its position each frame based on those values. You can attach this script to your sphere game object by dragging it onto the object in the Unity editor.
Working with Transforms and Animation
In Unity, transforms are used to manipulate the position, rotation, and scale of game objects. By working with transforms, you can create complex animations and movements that bring your game to life.
For example, let’s say you want to make your sphere move in a circular path around the center of the scene. You could do this by creating a new script that calculates the sphere’s position based on its current angle and speed:
csharp
using UnityEngine;
public class SphereAnimation : MonoBehaviour
{
public float radius = 5f; // how far from the center of the scene the sphere should move
public float speed = 10f; // how fast the sphere should move around the circle
public float angleSpeed = 360f / 60f; // how many degrees the sphere should turn per second
private Vector3 startPosition;
private float currentAngle = 0f;
void Start()
{
startPosition = transform.position;
}
void Update()
{
// calculate the x and y coordinates of the sphere’s position based on its angle
float x = Mathf.Sin(currentAngle) radius;
float y = Mathf.Cos(currentAngle) radius;
// move the sphere to the new position
transform.position = new Vector3(x, 0f, y);
// increment the angle and speed up the animation if it’s at full speed
currentAngle += angleSpeed * Time.deltaTime;
if (currentAngle > 360f)
{
currentAngle = 0f;
transform.position = startPosition;
}
}
}
This script calculates the sphere’s position based on its current angle and speed, and then moves it to the new position each frame. You can attach this script to your sphere game object and adjust the values of radius, speed, and angleSpeed to change the behavior of the animation.
Working with Materials and Lighting
In Unity, materials are used to give game objects a specific look and feel, such as making them shiny or transparent. By working with materials, you can create stunning visual effects that bring your game to life.
For example, let’s say you want to make a character look like it’s made of metal. You could do this by creating a new material that includes a metallic shader and applying it to the character object in the Unity editor:
csharp
using UnityEngine;
public class MetalMaterial : Material
{
public float shiny = 0.5f; // how shiny the metal should be (1 is completely shiny)
public Color color = Color.gray; // the color of the metal
private Shader _shader;
void OnEnable()
{
_shader = Shader.Find("Standard");
}
public Vector2 "_MainTex" ("_Main Tex", 2D) = "white" {}
public float "_Shiny" ("Shiny", Range) = shiny
public Color "_Color" ("Color", Color) = color
}
This material includes a shiny shader that controls how reflective the metal is, as well as a color parameter that lets you choose the color of the metal. You can apply this material to your character object by dragging it onto the object in the Unity editor.
In addition to materials, lighting is another important aspect of creating realistic visual effects