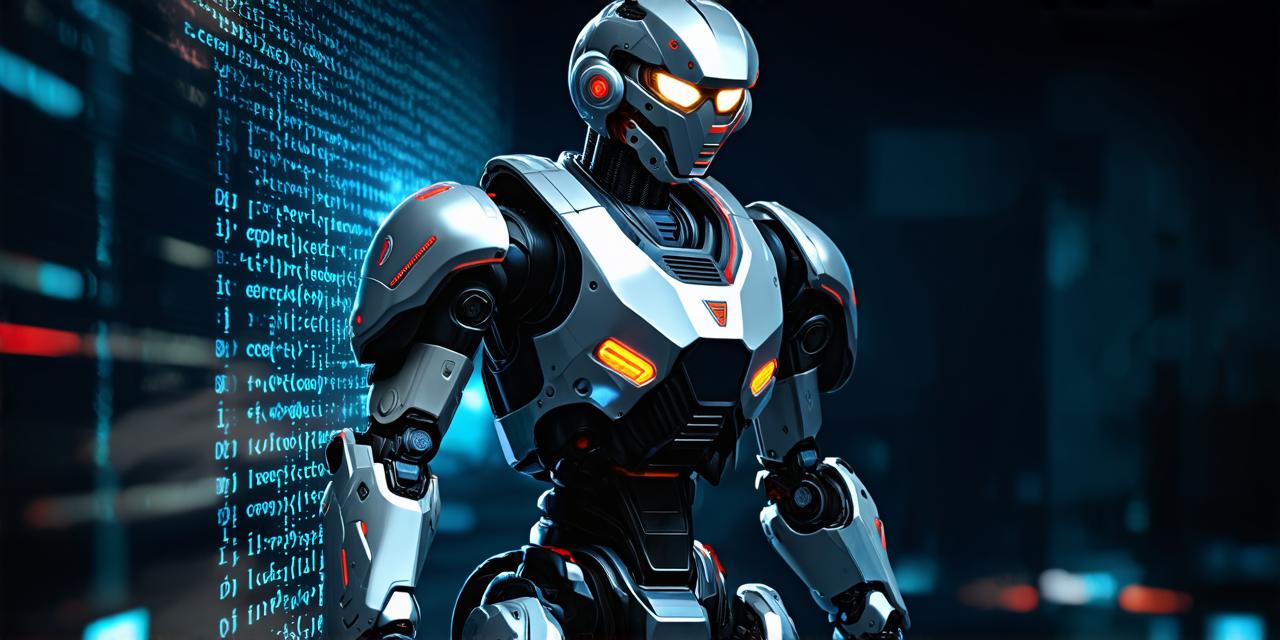
If you’re a Unity developer looking to add some excitement to your games, creating enemies that can target and shoot at players is a great way to increase engagement.
Before We Begin
Before we dive into the code, it’s important to understand some of the basic concepts involved in programming enemies in Unity. First, let’s take a look at some key terms:
- Player: The character that the player controls and interacts with in the game.
- Enemy: An NPC (non-playable character) or object that is designed to attack and defeat the player.
- Targeting: The process of identifying the player character and preparing to attack it.
- Shooting: The act of firing a weapon or projectile at the player.
Now that we have a basic understanding of these terms, let’s take a look at some key considerations when programming enemies in Unity:
- Difficulty Level: Enemies should be challenging but not too difficult for the player to defeat. You can adjust the difficulty level by adjusting factors such as enemy speed, health, and attack power.
- AI Behavior: Enemies should have some basic AI behavior, such as patrolling their territory or seeking out the player. You can use Unity’s built-in AI tools or third-party plugins to create more advanced AI behaviors.
- Weaponry: Enemies should be equipped with weapons that are appropriate for the game setting and difficulty level. You can create custom weapons or use pre-made assets from the Unity Asset Store.
- Visual Effects: Enemies should have visual effects such as animations and particle effects to make them more engaging and immersive.
Now that we have a basic understanding of these considerations, let’s take a look at how to program an enemy to target and shoot at the player in Unity.
Targeting the Player
The first step in programming an enemy to target and shoot at the player is to identify the player character in the game. You can do this by using Unity’s built-in GameObject.Find
method to locate the player’s game object.
Once you have located the player’s game object, you can use the Transform.position
property to get the player’s current position in the world. You can then use this position to calculate the direction and distance between the enemy and the player.
To target the player, you will need to create a script that will update the enemy’s aim and fire at the player. Here is an example script that demonstrates how to do this:
csharp
using UnityEngine;
public class EnemyShooter : MonoBehaviour
{
public GameObject projectilePrefab; // The prefab for the projectile
public float attackRange 10.0f; // The range at which the enemy can shoot
public float attackDamage 10.0f; // The damage dealt by the enemy’s attacks
private Transform playerTransform; // A reference to the player transform
private bool isAttacking false; // Whether the enemy is currently attacking the player
void Start()
{
// Find the player's game object
playerTransform GameObject.FindWithTag(“Player”).transform;
}
void Update()
{
// Check if the player is within attack range
if (Vector3.Distance(transform.position, playerTransform.position) < attackRange)
{
// If the player is within attack range, set the enemy's aim and fire at the player
isAttacking true;
transform.LookAt(playerTransform.position);
FireProjectile();
}
else
{
// If the player is not within attack range, stop attacking and reset the enemy's aim
isAttacking false;
transform.LookAt(Vector3.up);
}
}
void FireProjectile()
{
// Instantiate a new projectile at the enemy's position and fire it in the direction of the player
GameObject projectile Instantiate(projectilePrefab, transform.position, Quaternion.identity);
projectile.transform.LookAt(playerTransform.position);
}
}
This script uses the GameObject.Find
method to locate the player’s game object and the Vector3.Distance
method to check if the player is within attack range. If the player is within attack range, the script sets the enemy’s aim by looking at the player’s position using the transform.LookAt
method and then fires a projectile at the player using the Instantiate
method.
Once you have programmed the enemy to target the player, you will need to create a weapon or projectile that the enemy can use to attack the player. Here are some key considerations when creating a weapon in Unity:
- Weapon Type: There are many different types of weapons that you can create for your enemies, including ranged weapons such as guns and bows, and melee weapons such as swords and axes. Choose a weapon type that is appropriate for the game setting and difficulty level.
- Projectile Type: If you’re creating a ranged weapon, you will need to create a projectile that the enemy can fire at the player. The projectile should be designed to cause damage to the player and should have some basic physics properties such as speed, gravity, and friction.
- Weapon Animation: To make the weapon more engaging and immersive, you will need to create an animation for it. This animation can show the enemy firing the weapon or reloading it.
- Projectile Animation: If you’re creating a ranged weapon, you will also need to create an animation for the projectile. This animation can show the projectile flying through the air or exploding when it hits the player.
Once you have created your weapon and projectile, you will need to modify the EnemyShooter
script to use them. Here is an example of how to do this:
csharp
using UnityEngine;
public class EnemyShooter : MonoBehaviour
{
public GameObject projectilePrefab; // The prefab for the projectile
public float attackRange 10.0f; // The range at which the enemy can shoot
public float attackDamage 10.0f; // The damage dealt by the enemy’s attacks
private Transform playerTransform; // A reference to the player transform
private bool isAttacking false; // Whether the enemy is currently attacking the player
void Start()
{
// Find the player's game object
playerTransform GameObject.FindWithTag(“Player”).transform;
}
void Update()
{
// Check if the player is within attack range
if (Vector3.Distance(transform.position, playerTransform.position) < attackRange)
{
// If the player is within attack range, set the enemy's aim and fire at the player
isAttacking true;
transform.LookAt(playerTransform.position);
FireProjectile();
}
else
{
// If the player is not within attack range, stop attacking and reset the enemy's aim
isAttacking false;
transform.LookAt(Vector3.up);
}
}
void FireProjectile()
{
// Instantiate a new projectile at the enemy's position and fire it in the direction of the player
GameObject projectile Instantiate(projectilePrefab, transform.position, Quaternion.identity);
projectile.transform.LookAt(playerTransform.position);
}
}
This script uses the Instantiate
method to create a new projectile at the enemy’s position and fires it in the direction of the player by looking at the player’s position using the transform.LookAt
method. You will need to modify this code to use your own weapon and projectile prefab.
Summary
In this tutorial, we have learned how to create an enemy that can shoot at the player in a Unity game. We have also discussed some key considerations when creating weapons and projectiles for our enemies.