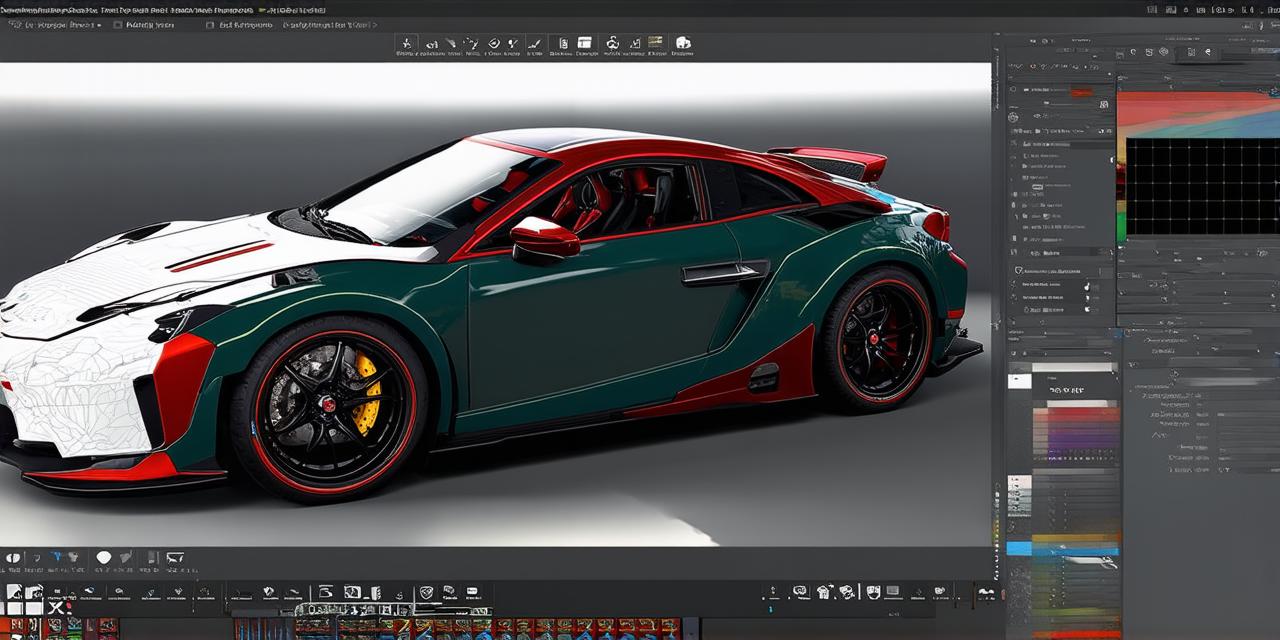
Unity is a powerful and versatile game engine that offers a vast range of features and capabilities. With its intuitive interface, Unity is an ideal platform for creating immersive and engaging games, virtual reality (VR) experiences, and interactive applications.
Introduction: Understanding Navigation in Unity 3D
Navigation is an essential aspect of creating any interactive application or game. It allows users to move through a virtual space, interact with objects, and access different areas of the application. In Unity 3D, there are several ways to implement navigation, including:
- Controllers
- Keyboard Input
- Touch Input
- Joystick Input
- Scene Management Techniques
1. Controllers
Controllers are perhaps the most popular way to navigate in Unity 3D. They offer a high level of precision and control over the player’s movement, making them ideal for first-person shooters (FPS) and other action-packed games.
Creating Custom Controllers
To create custom controllers in Unity, you can follow these steps:
- Open a new project in Unity.
- Navigate to the "Assets" menu and select "Create" > "User Interface."
- Drag a "Controller" object from the "UI" folder into the scene.
- In the Inspector window, click on the "Script" component of the controller object and add a new script.
- Name the script "Custom Controller" or any other name of your choice.
- Open the script in a text editor and write the following code:
using UnityEngine;
public class CustomController : MonoBehaviour
{
public float moveSpeed = 10f;
public Transform player;
private Vector3 movementInput;
void Update()
{
movementInput = new Vector3(Input.GetAxisRaw("Horizontal"), Input.GetAxisRaw("Vertical"), 0);
transform.position += movementInput * moveSpeed * Time.deltaTime;
}
}
7. Save the script and attach it to the controller object in the scene.
- In the Unity editor, select the player object and drag the controller object onto it as a child object.
Using Custom Controllers for Navigation
Once you have created your custom controllers, you can use them to navigate through your game world by adding additional script components and setting up scene management techniques.
Loading Scenes
To load scenes in Unity, you can use the `SceneManager` class. This class provides several methods for loading and unloading scenes, including:
LoadSceneAsync(int sceneIndex)
– loads a scene asynchronously, allowing you to continue executing other code while the scene is loading.LoadScene(string sceneName, LoadMode mode LoadMode.Single)
– loads a scene synchronously or asynchronously, depending on the value of themode
parameter.UnloadSceneAsync(int sceneIndex)
– unloads a scene asynchronously, allowing you to continue executing other code while the scene is unloading.UnloadScene(string sceneName, UnloadMode mode UnloadMode.All)
– unloads a scene synchronously or asynchronously, depending on the value of themode
parameter.
Camera Movement
The camera is an essential component of any game or application, as it allows players to view the game world from different angles and perspectives. In Unity, you can use several script components to control the movement of the camera, including:
CameraController
– a built-in script component that provides basic camera controls, such as zooming in and out, panning left and right, and tilting up and down.Custom Camera Controller
– a custom script component that allows you to create your own camera control scheme, such as using controllers or the mouse to move the camera.Cinematic
– a script component that allows you to create cinematic scenes, such as cutscenes and transitions between different scenes.
Object Interaction
Object interaction is an essential aspect of any game or application that requires players to interact with objects in the virtual space. In Unity, you can use several script components to enable object interaction, including:
Rigidbody
– a physics component that allows you to simulate the behavior of rigid bodies in your game world.Collider
– a physics component that defines the boundaries of an object and allows it to interact with other objects in the game world.Scriptable Object
– a special type of object in Unity that can contain scripts and data that are shared across multiple scenes and objects.Interactables
– specialized objects that allow players to interact with different elements in the game world, such as doors, switches, and levers.
Sound and Music
Sound and music are critical components of any interactive application or game, as they help to set the mood and atmosphere of the experience. In Unity, you can use several audio tools and techniques to add sound effects and music to your game or application, including:
Audio Source
– a script component that allows you to play