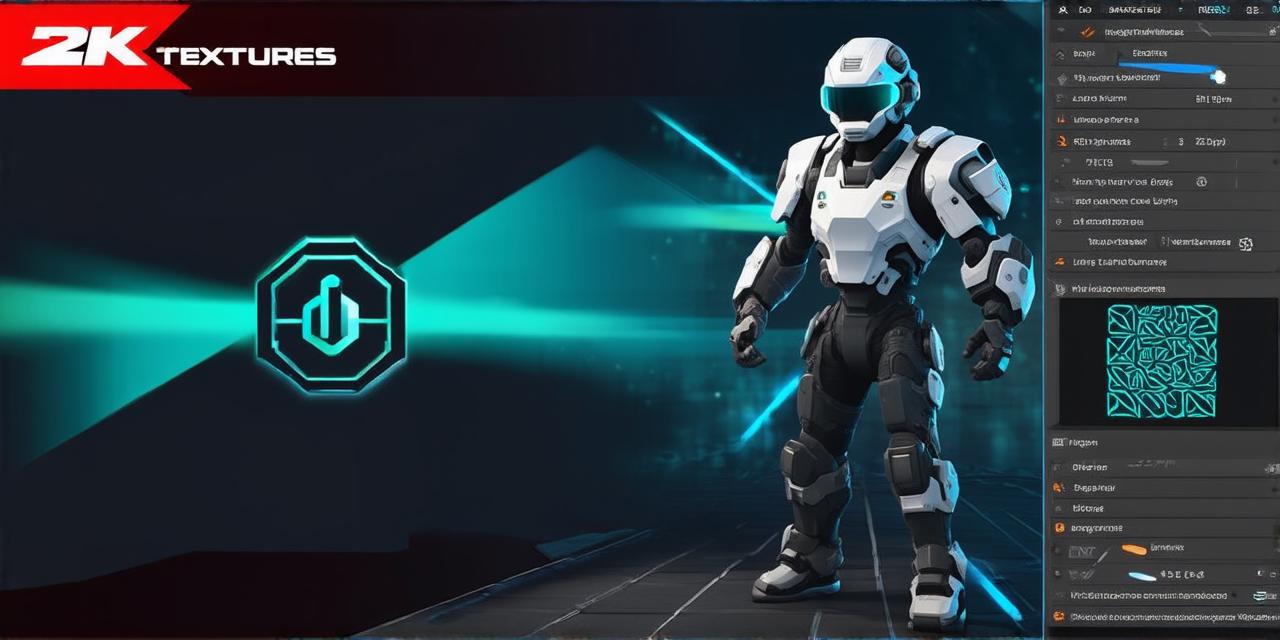
Making characters jump in a Unity game is a fundamental skill that every developer should have. In this article, we’ll walk you through the process of creating a jumping character in Unity step by step. We’ll also discuss some best practices and tips to help you optimize your character’s jump mechanics for maximum engagement and fun.
Step 1: Create a New Character Object
The first step to making a character jump in Unity is to create a new character object. You can do this by going to GameObject > 3D Object > Mesh Filter, then selecting the mesh you want to use for your character and adding it to the scene. Once you have your character object created, you can start adding components to it.
Step 2: Add Rigidbody and Animator Components
To make your character jump, you’ll need to add two essential components to your character object: a Rigidbody component and an Animator component. The Rigidbody component will allow you to control your character’s movement in the scene, while the Animator component will handle the character’s animations, including its jumps.
Step 3: Set Up the Jump Animation
Once you have added the Rigidbody and Animator components to your character object, it’s time to set up the jump animation. To do this, go to the Animator window in Unity and create a new animation clip for your character jumping. You can use any mesh that you like, but make sure it has a clear, distinct movement that represents a jump.
Step 4: Set Up the Jump Trigger
Next, you’ll need to set up a trigger for your character to jump. To do this, go to GameObject > Physics > Trigger and create a new trigger object in the scene. You can position the trigger wherever you like, but make sure it intersects with the ground plane or any other objects that your character will be jumping on.
Step 5: Set Up the Jump Script
Now it’s time to write the script that will control your character’s jump behavior. You can do this by going to Assets > Create > C Script, then opening the script in your favorite code editor. Here’s an example of what your jump script might look like:
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class CharacterJump : MonoBehaviour
{
public Transform groundCheck;
public float jumpForce = 10f;
void Update()
{
// Check if the player is pressing the jump button
if (Input.GetButtonDown("Jump"))
{
// Trigger the jump animation
animator.SetTrigger("Jump");
// Apply a force to make the character jump
rigidbody.AddForce(new Vector3(0f, jumpForce, 0f), ForceMode2D.Impulse);
}
}
}
Step 6: Adjust the Jump Parameters
Once you’ve written your jump script and set up the trigger and animation for your character, it’s time to adjust the parameters to get the desired results. For example, you can adjust the force applied by the Rigidbody component to control how high your character jumps. You can also adjust the timing of the jump animation to make it look more realistic or fun.
Best Practices for Jumping Characters in Unity
Here are some best practices to keep in mind when making characters jump in Unity:
- Make sure your character’s animations are clear and distinct, so players know what actions their character is performing.
- Use a trigger object to detect when your character is jumping, so you can apply the correct force and animation at the right time.
- Experiment with different jump heights and timings to create a fun and engaging jumping experience for your players.
- Make sure your character’s movement is smooth and fluid, so players don’t feel like they’re bouncing off walls or objects.
- Use particle effects or sound effects to enhance the overall experience of your game, making it more immersive and fun.
FAQs
Here are some frequently asked questions about jumping characters in Unity:
Q: How do I make my character jump higher?
A: You can increase the force applied by the Rigidbody component to make your character jump higher. You can also adjust the timing of the jump animation to give your character more time in the air.
Q: Why is my character’s jump not working?
A: Make sure you have added the necessary components, including a Rigidbody and Animator component, to your character object. Also, check that your trigger object is correctly positioned and that your jump script is correctly set up.
Q: How do I make my character’s jumping animation look more realistic?
A: You can experiment with different animations and timing to create a more natural-looking jumping motion for your character. You can also use particle effects or sound effects to enhance the overall experience of your game.
Conclusion
Making characters jump in Unity is an essential skill that every developer should have. By following these steps and best practices, you can create a fun and engaging jumping experience for your players. Remember to experiment with different parameters and effects to make your game stand out from the rest.