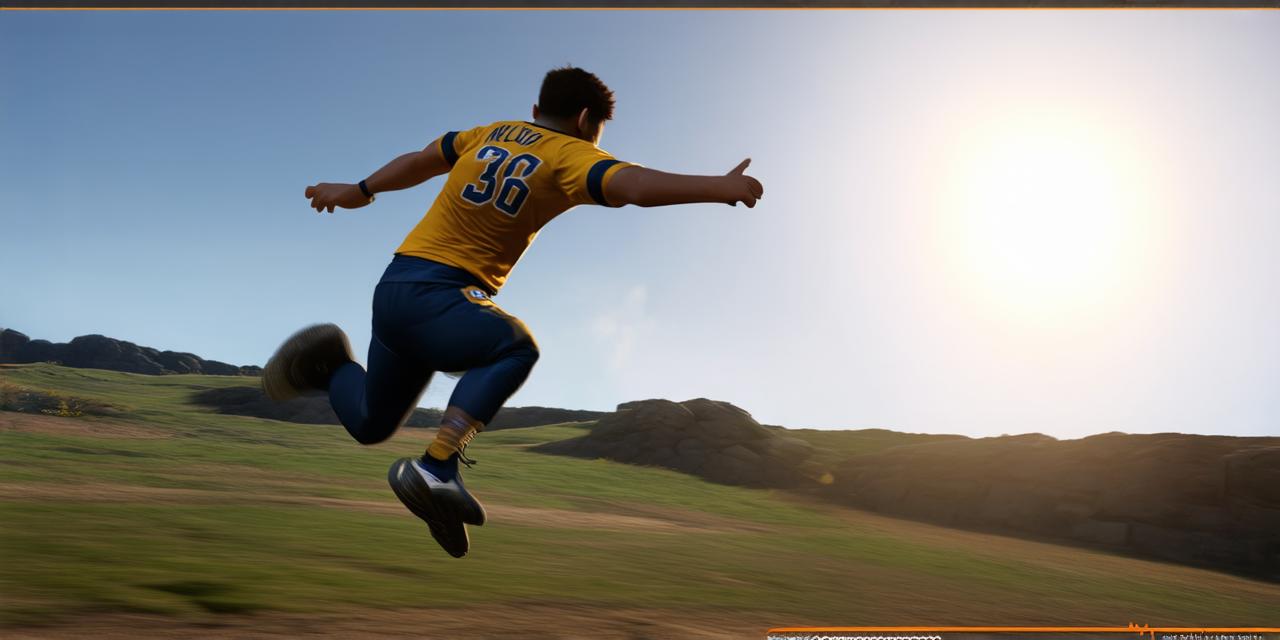
Jumping is one of the most basic and fundamental actions in any video game. It’s an action that players expect to be able to perform, and it’s an essential part of many gameplay mechanics. In this article, we will take a closer look at how to implement jumping in Unity 3D, including some best practices and tips for creating smooth, responsive, and engaging jumping mechanics.
Understanding the Basics of Jumping in Unity 3D
Before we dive into the implementation details, it’s important to understand the basics of jumping in Unity 3D. In order to jump, we need to define a few key components:
- Grounded: This is the state where the player character is touching the ground. When the player is grounded, they cannot jump.
- Jump force: This is the amount of force applied to the player character when they jump. The higher the jump force, the higher the player will jump.
- Jump height: This is the maximum distance that the player character can jump. This value depends on the jump force and the gravity in the scene.
- Jump animation: This is the animation that plays when the player character jumps. This animation gives feedback to the player that they have successfully jumped.
Implementing Jumping in Unity 3D
Now that we understand the basics of jumping let’s take a look at how to implement it in Unity 3D.
Setting Up Grounded Check
The first step is to set up the grounded check. This involves detecting when the player character is touching the ground and setting the grounded flag accordingly. We can use the built-in Physics.OverlapCircle
function to detect collisions with ground objects.
csharp
private void Update()
{
if (Input.GetButtonDown("Jump") && grounded)
{
Jump();
}
}
private void FixedUpdate()
{
CheckGrounded();
}
private void CheckGrounded()
{
Collider[] colliders = Physics.OverlapCircle(transform.position, 0.1f, LayerMask.GetMask("Ground"));
if (colliders.Length > 0)
{
grounded = true;
}
else
{
grounded = false;
}
}
Adjusting Jump Force and Height
The next step is to adjust the jump force and height values to create the desired level of difficulty and challenge for the player. We can do this by modifying the ForceMode2D
and Rigidbody2D
components attached to the player character object.
csharp
private void Jump()
{
rigidbody2D.AddForce(new Vector2(force, force), ForceMode2D.Impulse);
}
Implementing Smooth Transitions
To create a seamless and immersive experience for the player, we need to implement smooth transitions between grounded and airborne states. We can do this by using an animation controller and blending between different animations.
csharp
private void OnCollisionEnter2D(Collision2D collision)
{
if (collision.gameObject.CompareTag("Ground"))
{
grounded = true;
animator.SetBool("IsGrounded", true);
StartCoroutine(WaitForJump());
}
}
private IEnumerator WaitForJump()
{
yield return new WaitForSeconds(0.2f);
animator.SetBool("IsGrounded", false);
animator.SetInteger("State", 1); // Play jump animation
}
Adding Variety to Jump Mechanics
To keep the gameplay interesting and challenging, we can add variety to jump mechanics by including different types of jumps, such as wall jumps or double jumps. We can do this by modifying the Jump
function and adding new animations to the animation controller.
csharp
private void JumpWall()
{
rigidbody2D.AddForce(new Vector2(force Mathf.Cos(transform.localRotation.euler.x), force Mathf.Sin(transform.localRotation.euler.x)), ForceMode2D.Impulse);
}
private void DoubleJump()
{
rigidbody2D.AddForce(new Vector2(force 2, force 2), ForceMode2D.Impulse);
}
Testing and Iterating
Finally, it’s important to test our jump mechanics thoroughly and iterate on them based on feedback from players. We can do this by playing the game and observing how the player character moves and responds to different inputs. We can also use Unity’s built-in debugging tools to inspect the player character’s movement and force values.
csharp
Debug.Log(rigidbody2D.velocity); // Print the player character’s velocity
Conclusion
In this article, we have taken a closer look at how to implement jumping in Unity 3D. We have covered the basics of jumping, as well as some best practices and tips for creating smooth, responsive, and engaging jumping mechanics. By following these guidelines, you can create a fun and immersive gameplay experience that players will love.