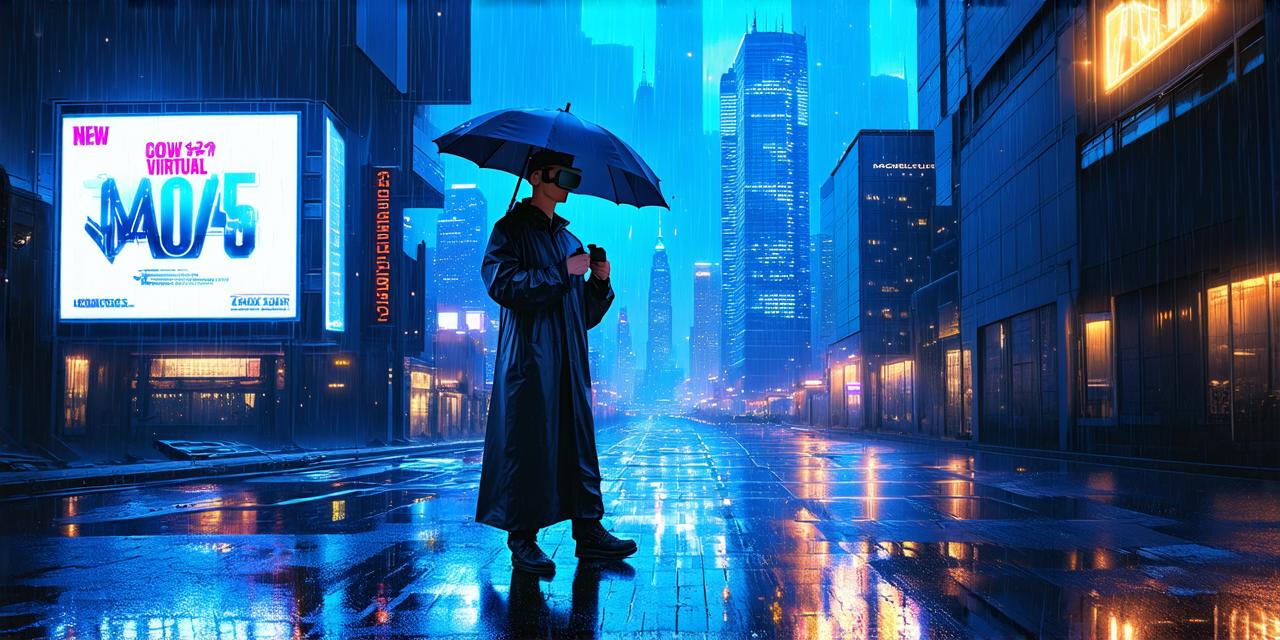
Corrected HTML code:
What is a Raycast?
A raycast is a technique used to detect collisions between objects in a 3D environment. It works by casting a line of light (or “ray”) from a given point in the scene and checking if it intersects with any other objects. If there is a collision, the raycast returns information about the object that was hit, such as its position and normal.
How to Implement a Raycast in Unity 3D
To implement a raycast in Unity 3D, you will need to use the built-in Physics.Raycast()
function. This function takes two arguments: the starting point of the ray and the direction it should travel. It returns information about the first collision that occurs along the ray, or false
if no collisions are found.
Optimizing Your Raycast Code
When working with raycasts in Unity 3D, it’s important to optimize your code for maximum performance. Here are some tips to help you do that:
Use a layer mask to filter which objects you want to check for collisions with. This can significantly improve the speed of your raycast by reducing the number of objects that need to be checked.
Use the
Physics.Raycast()
function'sTime
argument to control the speed of the ray. By setting this argument to a value greater than 0, you can make the ray cast slower and more accurate, which may be useful in certain situations.Avoid casting multiple rays at once, as each ray requires its own calculation and can slow down your code. Instead, use a single ray to cover as much of the scene as possible.
Use the
Physics.Raycast()
function'sLayerMask
argument to set a priority for different layers. This can help ensure that important objects (such as walls or obstacles) are checked before less important objects (such as background textures).Avoid using raycasts in situations where they may not be necessary, such as detecting collisions between small objects that are unlikely to intersect with each other. Instead, use other techniques such as bounding boxes or colliders.
Case Studies and Personal Experiences
As a Unity developer, I have used raycasts extensively in my projects. Here are a few examples of how they have been useful:
-
In a game where the player had to avoid obstacles by jumping over them, I used raycasts to detect collisions between the player and the obstacles. By checking for collisions at regular intervals, I was able to create a smooth and responsive gameplay experience.
-
In a puzzle game where the player had to solve puzzles by moving blocks around, I used raycasts to check for collisions between the blocks and other objects in the scene. This allowed me to create complex and challenging puzzles that required careful planning and timing.
-
In a virtual reality (VR) application where the user could interact with objects in the environment, I used raycasts to detect collisions between the user’s hands and the objects they were interacting with. This allowed me to create a realistic and immersive VR experience that felt like the user was truly inside the environment.
Conclusion
In conclusion, implementing a raycast in Unity 3D is a powerful tool for working with 3D environments. By using Physics.Raycast()
and optimizing your code for maximum performance, you can create interactive and engaging experiences that bring your projects to life. Whether you’re building a game, a VR app, or just a simple demo, raycasts are an essential part of any Unity developer’s toolkit.