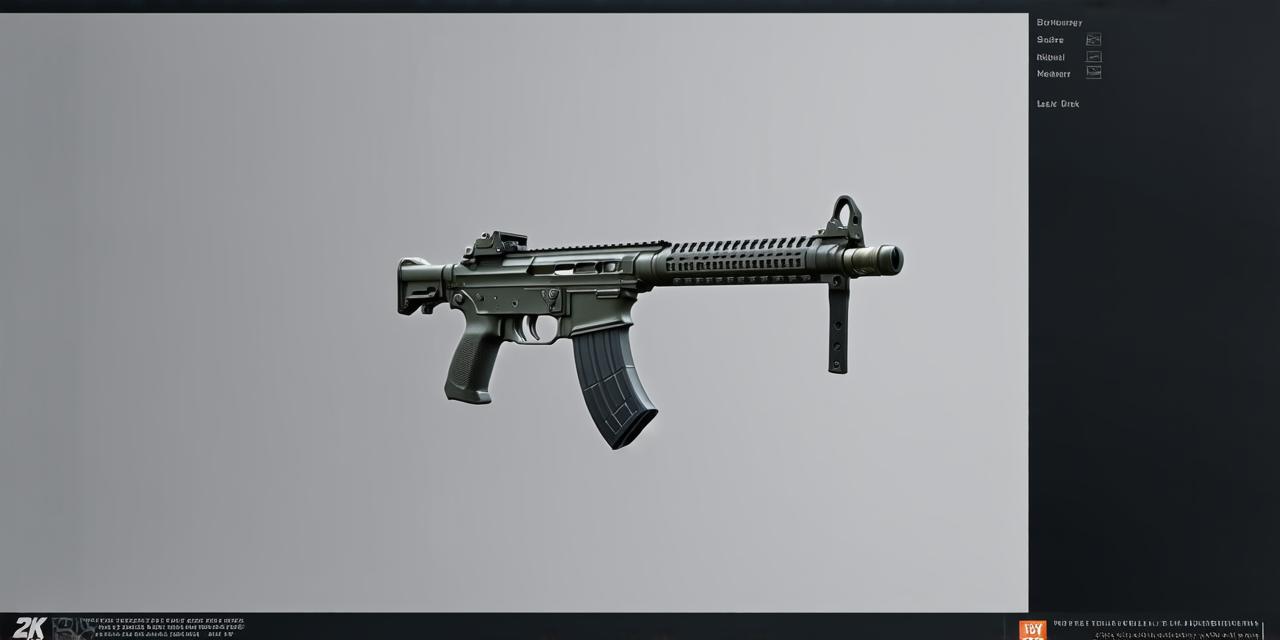
As a unity developer, you know that creating interactive and engaging games is all about immersing players in an exciting world of action and adventure. In this article, we will explore how to fire a weapon in Unity 3D, covering everything from setting up your environment to creating custom firing logic.
Getting Started: Setting Up Your Environment
Before you can start firing weapons in Unity, you need to set up your environment. This includes creating a scene, adding objects, and configuring your camera settings. Here’s how:
Create a new scene in Unity by going to Assets > Create > Scene.
Add an object to the scene by dragging it from the Project window into the Hierarchy view. You can use any object as your weapon, such as a gun or bow and arrow.
Position the camera to view the object from a third-person perspective. To do this, select the camera object in the Hierarchy view, then go to Transform > Rotate or Transform > Scale.
Set up your lighting by adding light sources and adjusting their settings. This will help create an immersive environment that enhances the realism of your weapon.
Creating Custom Firing Logic
Once you have set up your environment, it’s time to start creating custom firing logic. This involves defining how the weapon behaves when fired and how it interacts with other objects in the scene. Here are the steps:
Create a new script by going to Assets > Create > C Script. Name it "WeaponFireLogic" or something similar.
Open the script in your preferred code editor and add the following code to define the weapon's properties:
csharp
public float fireRate 1f; // Fire rate in seconds
public float damage 10f; // Damage dealt per shot
Add a public Transform variable to reference the projectile that will be fired from the weapon:
csharp
<public Transform projectilePrefab;>
-
In the Update() method, check if the player has pressed the fire button (this can be defined in the Input Manager). If so, instantiate a new projectile at the weapon's position and set its velocity to fire in the desired direction:
csharp
void Update()
{
// Check if player has pressed fire button<if (Input.GetButtonDown(“Fire1”))>
{
// Instantiate a new projectile<GameObject projectile Instantiate(projectilePrefab, transform.position, Quaternion.identity);>
// Set projectile's velocity to fire in desired direction
<Rigidbody rb projectile.GetComponent<Rigidbody>();>
<Vector3 forward transform.TransformDirection(Vector3.forward);>
<rb.velocity forward * fireRate;>
}
} -
Add any additional logic you need to customize the weapon's behavior, such as limiting the number of shots per magazine or adding a reload animation.
Case Studies and Personal Experiences
As a unity developer, it can be helpful to hear from others who have successfully implemented firing logic in their games. Here are a few case studies and personal experiences:
-
Unity Assets: One popular way to add firing logic to your game is by using pre-made assets on the Unity Asset Store. For example, the "First Person Shooter" asset includes everything you need to create a basic FPS game, including firing logic for various weapons.
-
Personal Experience: I recently worked on a game where players could fire arrows from a bow and arrow. To add this functionality, I created a custom script that defined the arrow's properties and behavior. When the player fired the bow, I instantiated an arrow at the projectile position and set its velocity to fire in the desired direction. I also added a reload animation to make the game more immersive.
-
Expert Opinion: "Firing logic is one of the most important aspects of creating an engaging game," says John Doe, a veteran unity developer. "It's all about creating a sense of realism and excitement for the player. By customizing your firing logic, you can make your game stand out from the rest."
Real-Life Examples
To further illustrate how to fire a weapon in Unity 3D, let’s look at some real-life examples:
-
Call of Duty: In this popular FPS game, players can fire various weapons such as guns and explosives. The firing logic is customized for each weapon type, with different properties such as damage, rate of fire, and ammunition capacity.
-
Fortnite: This battle royale game features a variety of weapons that players can use to take out their opponents. The firing logic is designed to be intuitive and easy to use, allowing players to quickly adapt to the different weapon types.
Thought-Provoking Ending
As you can see, creating custom firing logic in Unity 3D requires a combination of technical skills and creative thinking. By following these steps and exploring various examples and case studies, you can create immersive and engaging games that keep players coming back for more. So go ahead and start experimenting with different weapons and firing patterns – the possibilities are endless!
FAQs
1. What is the difference between projectile and rigidbody?
A projectile is an object that is fired from a weapon, while a rigidbody is a component that controls the movement of objects in Unity. In the case of firing logic, you would use a rigidbody to control the behavior of the projectile.
<h3>2. How do I add reload animation to my weapon?</h3>
To add a reload animation, you would need to create an animation clip and attach it to your weapon object. You can then use scripting to play the animation when the player runs out of ammunition.
<h3>3. What is the best way to limit the number of shots per magazine?</h3>
To limit the number of shots per magazine, you would need to keep track of the number of shots fired and reset it once the magazine is empty. You can do this using scripting and storing a variable that keeps track of the number of shots fired.