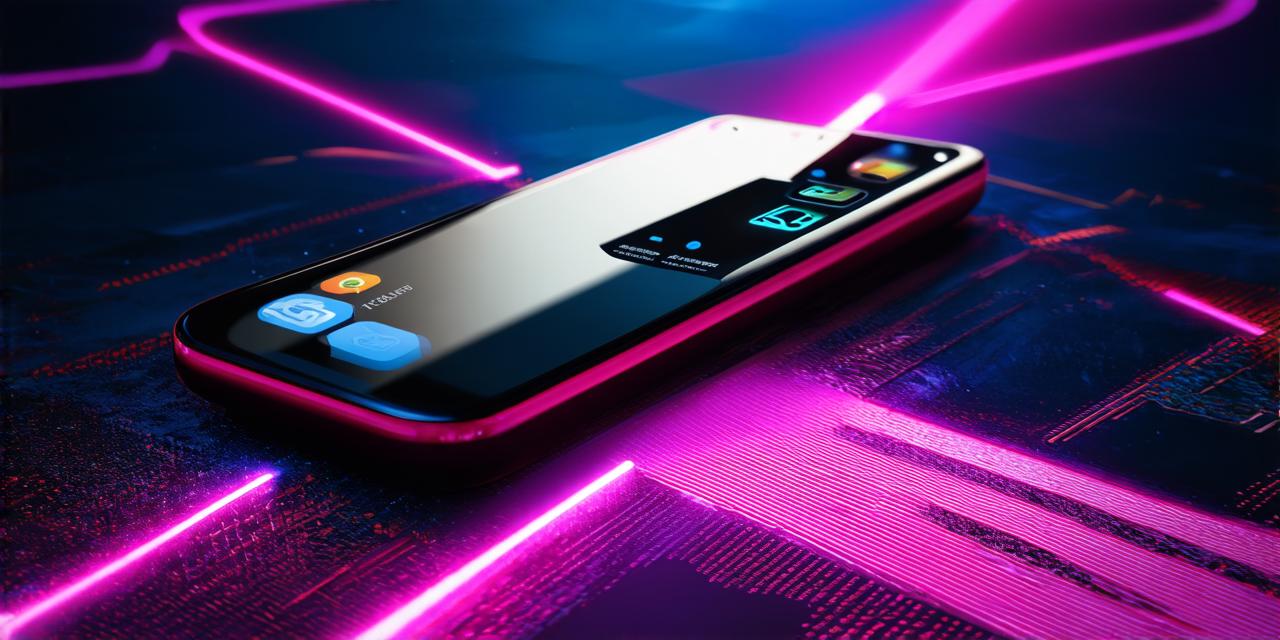
<p>Introduction</p>
Augmented reality (AR) is becoming increasingly popular in various industries, including gaming, marketing, and education. With the help of AR, users can interact with virtual objects in the real world, providing a unique and immersive experience. In this guide, we will explore how to develop an augmented reality application using Unity 3D, one of the most popular game engines on the market.
What is Unity 3D?
Unity 3D is a cross-platform game engine that allows developers to create 2D and 3D games, as well as virtual reality (VR) and augmented reality (AR) experiences for various devices such as smartphones, tablets, computers, and consoles. Unity 3D supports C scripting, which is a powerful programming language that allows for easy scripting of AR applications.
Getting Started with Unity 3D
Before diving into the development process, it’s important to set up your development environment. First, download and install Unity Hub, which is a tool that simplifies the installation of Unity and other related software. Once installed, open Unity Hub and select "AR" as the project template. This will create a new AR project with all the necessary assets and configurations.
Next, create a new scene in your AR project by right-clicking on the Hierarchy window and selecting "Create Empty." Name your scene something descriptive like "AR Scene." Now, you can start adding objects to your scene, either by importing them from asset stores or by creating them yourself using 3D modeling software.
Designing Your AR Experience
Once you have your scene set up, it’s time to design your AR experience. AR experiences typically involve interacting with virtual objects in the real world. To achieve this, you will need to create a marker object that triggers the AR experience when scanned by a device’s camera.
To create a marker object, go to the "Assets" window and select "Create > 3D Object." Name your object something descriptive like "Marker." Now, select your marker object in the Hierarchy window and go to the "Renderer" component in the Inspector window. Here, you can add a texture to your marker object that will be recognized by the AR device’s camera.
Once you have your marker object set up, you can start adding objects to your scene that will interact with it. For example, you might create a 3D model of a product that users can examine in augmented reality when they scan the marker object. To add this object to your scene, go to the "Assets" window and select "Import Package."
Implementing AR Functionality
Now that you have your scene set up with objects and markers, it’s time to implement the AR functionality. To do this, you will need to create a script that will be attached to your marker object. This script will handle the interaction between the real world and the virtual world.
Open your script in a code editor and add the following code:
javascript
using UnityEngine;
using System.Collections;
public class ARInteraction : MonoBehaviour
{
public GameObject virtualObject;
void Start()
{
// Set the virtual object to be invisible by default
virtualObject.SetActive(false);
}
void Update()
{
// Check if the marker is within the camera’s field of view
if (transform.localScale.x > 0.5f && transform.localScale.z > 0.5f)
{
// Set the virtual object to be visible when the marker is within the camera’s field of view
virtualObject.SetActive(true);
}
else
{
// Set the virtual object to be invisible when the marker is outside the camera’s field of view
virtualObject.SetActive(false);
}
}
}
This script checks if the marker object is within the camera’s field of view and sets the virtual object to be visible or invisible accordingly. Now, attach this script to your marker object in the Unity editor and import your virtual object into the scene.
Testing Your AR Application
Now that you have implemented the AR functionality, it’s time to test your application. To do this, connect your device to your computer and open the Unity editor. Select "Build Settings" from the top menu bar and select the appropriate platform for your target device (e.g., Android or iOS). Then, click on the "Build" button to generate an executable file for your target device.
Once you have generated the executable file, run it on your device and scan the marker object with your device’s camera. The virtual object should appear in augmented reality, allowing you to interact with it in real time.
Case Study: AR App Development Using Unity 3D
One great example of an AR app developed using Unity 3D is the popular game "Pokemon Go." This game uses AR technology to allow players to catch virtual creatures in the real world. The game has been a huge success, with millions of users worldwide playing it.
The development team at Niantic, the company behind Pok