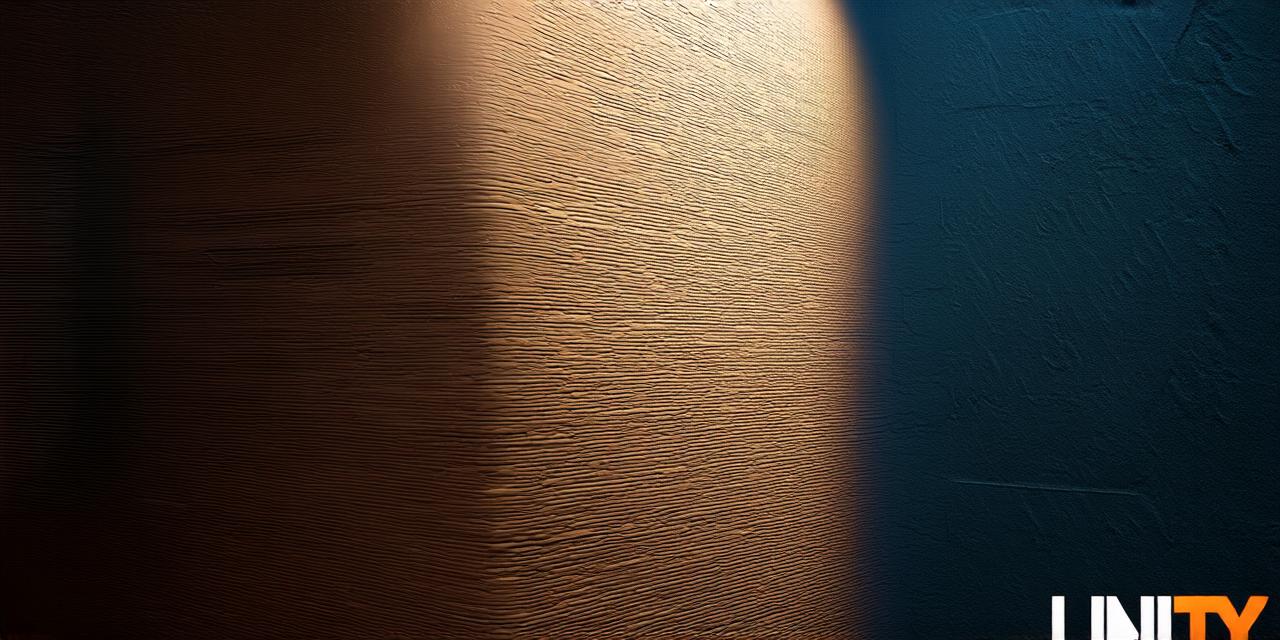
Building walls in Unity 3D can be a daunting task for beginners and experienced developers alike. However, with the right knowledge and tools, anyone can create stunning, realistic walls that enhance the overall look and feel of their Unity projects.
Creating Walls with Meshes
One of the most common ways to create walls in Unity 3D is by using meshes. A mesh is a 3D model that can be imported into Unity and then manipulated to create various shapes and forms, including walls. To create a wall using a mesh, follow these steps:
-
Import the mesh: First, you need to import the mesh into Unity. You can do this by clicking on "Assets" in the menu bar at the top of the screen, then selecting "Import Package" and choosing the mesh file you want to use.
-
Position and scale the mesh: Once the mesh is imported, you need to position and scale it to fit the space where you want the wall to be. To do this, select the mesh in the Hierarchy view, then use the Transform tool to move and rotate it as needed. You can also use the Scale tool to adjust the size of the mesh.
-
Add textures: To give your wall a realistic look, you need to add textures to it. Textures are images that are applied to the surface of the mesh to give it color and detail. To add a texture, select the mesh in the Hierarchy view, then click on "Material" in the Inspector window on the right side of the screen. From there, you can drag and drop textures onto the material to apply them to the mesh.
-
Add lighting: Lighting is crucial for creating a realistic environment in Unity. To add lighting to your wall, select the wall in the Hierarchy view, then click on "Lighting" in the menu bar at the top of the screen. From there, you can adjust the lighting settings to create the desired effect.
Creating Walls with Scripts
Another way to create walls in Unity 3D is by using scripts. A script is a piece of code that can be attached to a game object in Unity to control its behavior. To create a wall using a script, follow these steps:
-
Create a new script: First, you need to create a new script in Unity. You can do this by clicking on "Assets" in the menu bar at the top of the screen, then selecting "Create" and choosing "C Script."
-
Write the script code: In the script editor that appears, you need to write the code for your wall. The exact code will depend on the specific wall you want to create, but here is an example of a simple wall script:
scss
using UnityEngine;
public class Wall : MonoBehaviour {
public float wallHeight = 10f; // height of the wall
public float wallWidth = 10f; // width of the wall
public Vector3 startPosition = new Vector3(0f, 0f, 0f); // position of the wall to start at
public Vector3 endPosition = new Vector3(10f, 0f, 0f); // position of the wall to end at
public Material wallMaterial; // material of the wall
private void Start() {
Vector3 startPoint = transform.position;
Vector3 direction = (endPosition – startPosition) / wallWidth;
Transform wallTransform = Instantiate(wallPrefab, startPosition + direction * 0.5f, Quaternion.identity);
wallTransform.SetParent(transform);
wallTransform.localScale = new Vector3(wallWidth, wallHeight, wallWidth);
wallTransform.position = startPoint;
}
} -
Attach the script to a game object: Once you have written the script code, you need to attach it to a game object in Unity. To do this, select the game object in the Hierarchy view, then click on "Add Component" in the Inspector window and choose "Script." Drag and drop the wall script into the component.
-
Set up the prefab: Before you can use the script, you need to create a prefab of the wall. To do this, select the wall mesh in the Scene view, then go to "Edit" > "Select All" in the menu bar at the top of the screen. Right-click on an empty area and choose "Create" > "Prefab." Name the prefab "Wall Prefab" and drag it into the Assets folder in the Project window.
-
Set up the wall object: Finally, you need to create a game object for the wall. To do this, go to "GameObject" > "3D Object" > "Cube" in the menu bar at the top of the screen. Name the cube "Wall" and drag it into the Scene view. Select the cube in the Hierarchy view, then go to "Inspector" > "Prefab" in the menu bar at the top of