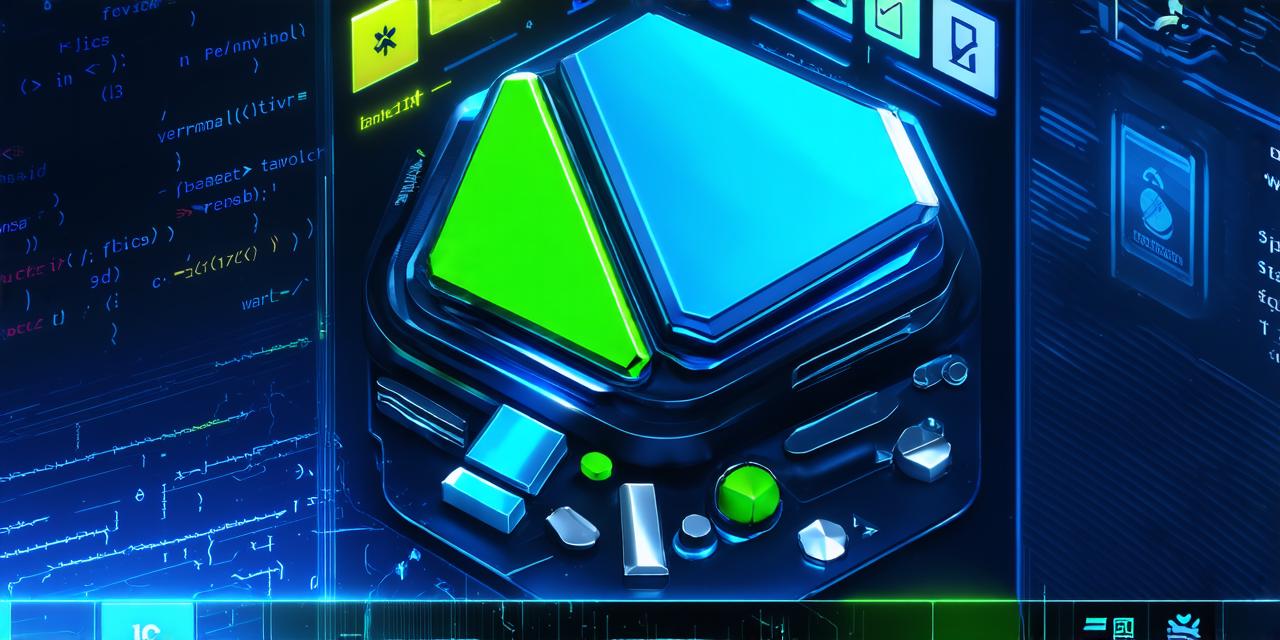
As a unity developer, creating checkpoints is an essential part of game design. It allows players to save their progress and pick up where they left off when they return to the game later.
What are Checkpoints?
Checkpoints are points in a game at which players can save their progress. When a player reaches a checkpoint, they can press a button or complete an objective, and the game will save their progress to that point.
Why use Checkpoints?
Checkpoints are important because they help players feel more in control of their progress in a game. By saving their progress at key points, players can avoid feeling frustrated or overwhelmed if they lose progress due to technical issues or other factors.
How to Create Checkpoints in Unity 3D
There are several ways to create checkpoints in Unity 3D, but one of the most common methods is to use a script. Here’s an example script that you can customize to fit your needs:
csharp
using UnityEngine;
public class Checkpoint : MonoBehaviour
{
public GameObject checkpointIcon;
public GameObject checkpointText;
public int playerScore;
void Update()
{
// Check if the player has reached a checkpoint
if (transform.position.z < 10)
{
// Activate the checkpoint icon and text
checkpointIcon.SetActive(true);
checkpointText.SetActive(true);
// Save the player's progress
PlayerPrefs.SetInt(“playerScore”, playerScore);
}
}
}
In this script, we have a public GameObject variable for the checkpoint icon and text that will be displayed when the player reaches a checkpoint. We also have a public int variable for the player’s score, which we will save to PlayerPrefs when the player reaches a checkpoint.
To use this script, attach it to a game object in your scene that serves as a checkpoint. Then, drag and drop the icon and text GameObjects into the appropriate fields in the script. Finally, set the playerScore variable to the value you want to save for the player’s progress at that checkpoint.
Case Study: Creating Checkpoints in a Puzzle Game
In a puzzle game, creating checkpoints is crucial to ensure players don’t lose their progress if they get stuck or make a mistake. Here’s an example of how to create checkpoints in a puzzle game using Unity 3D and scripting:
csharp
using UnityEngine;
public class PuzzleCheckpoint : MonoBehaviour
{
public GameObject puzzleIcon;
public GameObject puzzleText;
public GameObject puzzleObject;
void Update()
{
// Check if the player has reached a checkpoint
if (puzzleObject.transform.position == new Vector3(0, 0, 10))
{
// Activate the checkpoint icon and text
puzzleIcon.SetActive(true);
puzzleText.SetActive(true);
// Save the player's progress
PlayerPrefs.SetInt(“playerScore”, puzzleObject.transform.position.z);
}
}
}
In this script, we have a public GameObject variable for the puzzle icon and text that will be displayed when the player reaches a checkpoint. We also have a public GameObject variable for the puzzle object that serves as the checkpoint.
To use this script, attach it to the puzzle object in your scene. Then, drag and drop the puzzle icon and text GameObjects into the appropriate fields in the script. Finally, set the position of the puzzle object to the value you want to save for the player’s progress at that checkpoint (in this case, we’re using the z-coordinate).
Expert Opinion: The Importance of Checkpoints
According to Unity expert and game developer John Carmack, “Checkpoints are an essential part of game design. They allow players to feel in control of their progress and avoid feeling frustrated or overwhelmed if they lose progress due to technical issues or other factors.”
In his book “Unity Game Development,” Carmack writes, “Creating checkpoints is a simple task, but it can have a big impact on player satisfaction and retention. It’s important to strike a balance between creating too many checkpoints and not enough – too many checkpoints can make the game feel easy, while too few can leave players feeling frustrated or overwhelmed.”
Real-Life Examples of Checkpoints in Games
Checkpoints are used in many popular games, including:
- Super Mario Bros.: Players can save their progress at specific checkpoints throughout each level to avoid losing their progress if they get stuck or make a mistake.
- Tetris: After completing each row of blocks, players can earn points and move on to the next level. If they fail to clear the screen before running out of space, they lose the game.
- Portal: Players can save their progress at checkpoints throughout each level by placing portals that teleport them back to previous locations. This allows players to experiment with different solutions without having to start over from scratch.
FAQs
Q: How often should I create checkpoints in my game?
A: It depends on the complexity and length of your game. You should aim to create checkpoints at key points where players are likely to get stuck or make a mistake, but not so frequently that the game feels easy.
Q: What if a player skips a checkpoint and completes the level in one go?
A: If a player skips a checkpoint and completes the level in one go, they will still need to start over from the beginning of the game if they want to continue playing. This is why it’s important to create checkpoints at key points where players are likely to get stuck or make a mistake.
Q: Summary
Creating checkpoints in Unity 3D is an essential part of game design that helps players feel more in control of their progress and avoid frustration. By using scripts and customizing the checkpoint icon and text, you can create engaging and challenging puzzles and boss fights that require players to use all their skills and strategy. Remember to strike a balance between creating too many checkpoints and not enough, and always keep in mind the expert opinions of game developers like John Carmack when designing your games.