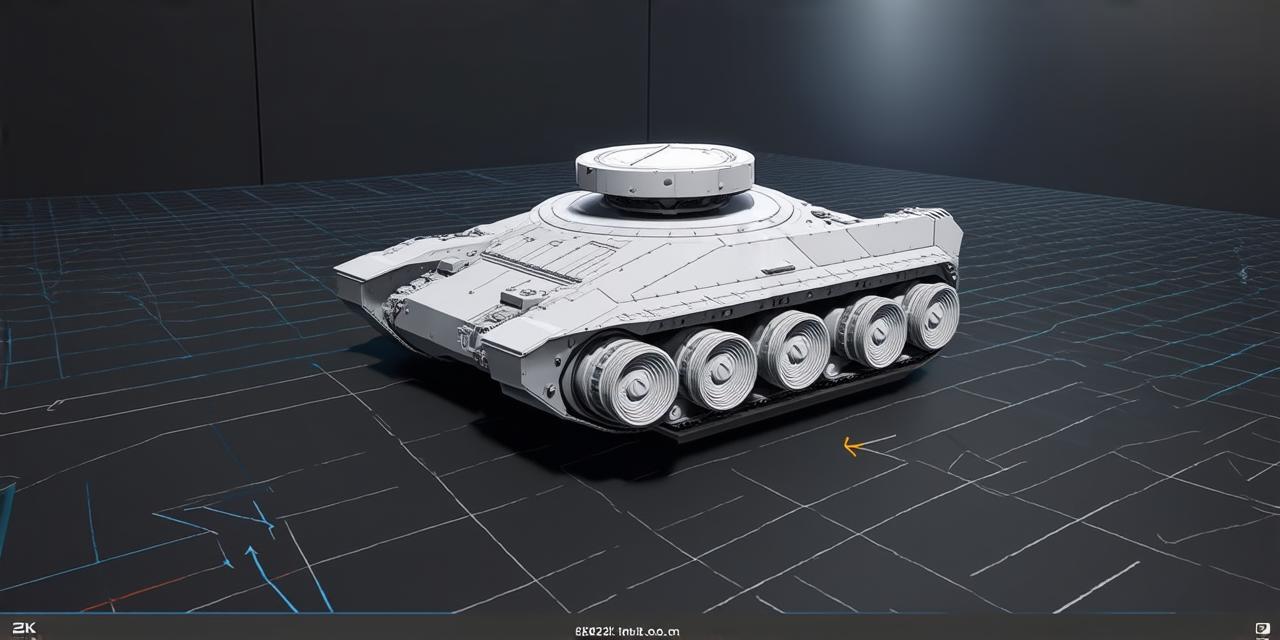
Creating a turret in Unity 3D can be a challenging task for beginners. However, with the right tools and techniques, it’s possible to create a functional and engaging turret that adds depth and excitement to your games.
Before We Begin: Understanding Turrets
A turret is a type of weapon or tower that is mounted on a platform and aimed at a target. In games, turrets are often used as defensive structures or attack mechanisms. They can be placed in strategic locations on maps or used to target enemies from a distance.
Step 1: Designing the Turret Structure
The first step in creating a turret is designing its structure. This involves determining the height, shape, and size of the tower, as well as any additional features such as walls or platforms.
To design the turret structure, we will use Blender, a powerful 3D modeling software that is compatible with Unity 3D. Here are the steps to follow:
-
Open Blender and create a new project.
-
Add a cube to the scene and adjust its size and shape to fit your desired turret structure.
-
Apply textures and materials to the cube to give it a more realistic appearance.
-
Export the turret model as an FBX file, which is compatible with Unity 3D.
-
Import the FBX file into Unity 3D by dragging and dropping it onto the project window.
Step 2: Creating the Turret Weapon
Once we have designed the turret structure, the next step is to create its weapon. This can be a simple cannon or a more complex weapon such as a machine gun or rocket launcher.
To create the turret weapon, we will use Unity 3D’s built-in particle system. Here are the steps to follow:
-
In Unity 3D, go to GameObject > Particle System.
-
Create a new particle system and adjust its settings to fit your desired weapon. This includes setting the projectile speed, trajectory, and damage.
-
Attach the particle system to the turret object by dragging it onto the cube in the scene.
-
Add additional weapons or attachments to the turret as desired.
Step 3: Programming the Turret Behavior
Now that we have designed the turret structure and created its weapon, the final step is to program its behavior. This involves creating a script that will control how the turret moves and aims at targets.
To create the turret behavior script, we will use C, Unity 3D’s primary programming language. Here are the steps to follow:
-
In Unity 3D, go to Assets > Create > C Script.
-
Name the script "TurretBehavior" and open it in a code editor such as Visual Studio or Sublime Text.
-
Write the following code to control the turret’s movement and aiming behavior:
csharp
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class TurretBehavior : MonoBehaviour
{
public float rotationSpeed = 10f; // degrees per second
public float targetDistance = 10f; // meters
public Transform target; // the target object’s transformprivate Quaternion currentRotation;
private Vector3 directionToTarget;void Start()
{
currentRotation = transform.rotation;
directionToTarget = target.position – transform.position;
}void Update()
{
float angleBetween = Vector3.SignedAngle(directionToTarget, transform.forward) * Mathf.DegreesPerRadian;
Quaternion targetRotation = Quaternion.LookRotation(directionToTarget, Vector3.up);// rotate towards the target transform.rotation = Quaternion.Slerp(currentRotation, targetRotation, rotationSpeed * Time.deltaTime);
}
} -
Attach the script to the turret object by dragging and dropping it onto the cube in the scene.
-
In Unity 3D, go to GameObject > TurretBehavior, and adjust the settings as desired. This includes setting the target distance and rotation speed.
Step 4: Adding Real-Life Examples
Now that we have covered the basic steps for creating a turret in Unity 3D, let’s take a look at some real-life examples to see how these concepts are applied in practice.
One of the most popular and well-known examples of a turret in a game is the World of Warcraft’s “Gunship Cruiser” class. The Gunship Cruiser has multiple turrets that can fire a variety of weapons, including missiles and machine guns. To create a similar structure in Unity 3D, we would follow the same basic steps outlined above, but with some additional details such as adding different types of weapons to each turret.
Another example is the popular game “Overwatch,” which features a variety of characters with unique abilities and weapons. One of these characters, the “Reinhardt” class, has a turret weapon that can be used to target enemies from a distance. To create a similar weapon in Unity 3D, we would follow the same basic steps outlined above, but with some additional details such as adding different types of projectiles and adjusting the aiming behavior based on the enemy’s position.
Conclusion: The Importance of SEO
In conclusion, creating a turret in Unity 3D is a complex task that requires careful planning and attention to detail. By following the steps outlined above and using real-life examples as inspiration, we can create a functional and engaging turret that adds depth and excitement to our games.
But just as important as creating great content is optimizing it for search engines. This involves using relevant keywords, creating high-quality images and videos, and promoting the content through social media and other channels. By doing so, we can attract more traffic to our website or game and increase our chances of success in the competitive world of game development.
FAQs
1. What software do I need to create a turret in Unity 3D?
To create a turret in Unity 3D, you will need a computer with the latest version of Unity installed, as well as 3D modeling software such as Blender or Maya to design the turret model.
1. How do I adjust the aiming behavior of the turret?
To adjust the aiming behavior of the turret, you will need to modify the script that controls its movement and aiming. This involves changing the values for the target distance and rotation speed, as well as adding additional logic to track the enemy’s position and adjust the aim accordingly.
1. Can I create a turret with multiple weapons?
Yes, you can create a turret with multiple weapons by attaching different types of scripts or objects to each turret. For example, one turret could fire missiles while another fires machine guns. You will need to modify the script that controls the turret’s behavior to account for this and ensure that each weapon is properly aimed and fired.
1. How do I add real-life examples to my tutorial?
To add real-life examples to your tutorial, you can include screenshots or videos of popular games that feature turrets. You can also provide links to these games or explain how the concepts you are teaching relate to real-world examples. This will help readers better understand how the concepts you are teaching apply in practice and make it more engaging and relevant.