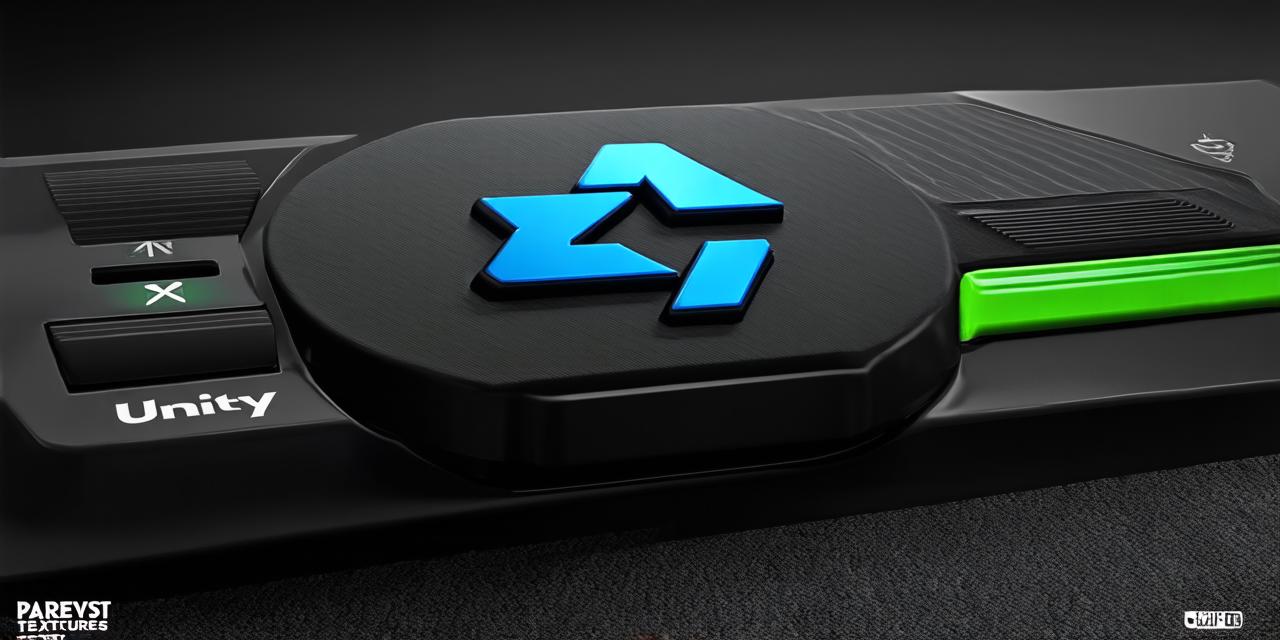
In this comprehensive guide, we will walk you through the process of creating buttons in Unity 3D step-by-step. We’ll cover everything from setting up the button prefab to scripting and customizing the button’s appearance.
Creating the Button Prefab:
The first step in creating a button in Unity 3D is to set up the prefab. A prefab is a reusable object that can be saved and loaded into multiple scenes. To create a button prefab, follow these steps:
-
In the Unity editor, navigate to
Assets > Create > UI > Button (Scripted).
-
Drag and drop the button onto the canvas.
-
In the Inspector window, give your button a name and set its size and position.
-
Open the Script tab and add a new C script to the button. Name it “ButtonScript”.
-
Attach the ButtonScript to the button by dragging it from the Project window onto the button in the Hierarchy view.
Scripting the Button:
Now that you have your button prefab set up, it’s time to start scripting. The ButtonScript is where we will add the functionality to our button. Here are the steps to create a basic button script:
-
Open the ButtonScript in your favorite text editor.
-
Add the following code to the OnClick() function:
-
Save the script and attach it to the button in the Unity editor.
-
In the Inspector window, add a new public GameObject variable to the button script. Name it “Target”. This will be the object that the button will interact with when clicked.
-
Drag and drop the Target object onto the button in the Hierarchy view.
-
Now you can add the code to execute when the button is clicked inside the OnClick() function. For example, you could change the color of the target object or play an animation.
csharp
public void OnClick()
{
// Code to execute when the button is clicked goes here.
}
Customizing the Button’s Appearance:
Once your button is functional, you may want to customize its appearance. Unity 3D offers a wide variety of options for customizing buttons, including changing the texture, font, and size. Here are some tips for customizing your button’s appearance:
-
Use the Image component to change the texture of the button. You can find the Image component in the Assets > UI > Image folder.
-
Use the Text component to add text to the button. You can find the Text component in the Assets > UI > Text folder.
-
Use the RectTransform component to adjust the size and position of the button. You can find the RectTransform component in the Assets > UI > Layout folder.
-
Experiment with different fonts and colors to create a unique look for your button.
Creating a Button with Multiple Interactions:
If you want to create a button that has multiple interactions, you can do so by adding additional OnClick() functions to the ButtonScript. For example, you could have one OnClick() function that changes the color of an object and another that plays an animation. To add multiple interactions, simply create new OnClick() functions and assign them to different actions.
Creating a Button that Interacts with Other Objects in the Scene:
To create a button that interacts with other objects in the scene, you need to assign a Target object to the button script. The Target object is the object that the button will interact with when clicked. To do this, follow these steps:
-
In the Unity editor, navigate to the Hierarchy view and select the button object.
-
In the Inspector window, add a new public GameObject variable to the button script. Name it “Target”.
-
Drag and drop the Target object onto the button in the Hierarchy view.
-
Now you can assign the Target object to the button script by dragging it from the Project window onto the Target variable in the Inspector window.
-
You can now add code to execute when the button is clicked that interacts with the Target object. For example, you could change the position of the Target object or destroy it.
Conclusion:
In conclusion, creating buttons in Unity 3D is a straightforward process that can be done using prefab setup, scripting, and customization. By following the steps outlined in this guide, you should have no problem creating functional and visually appealing buttons for your 3D projects.