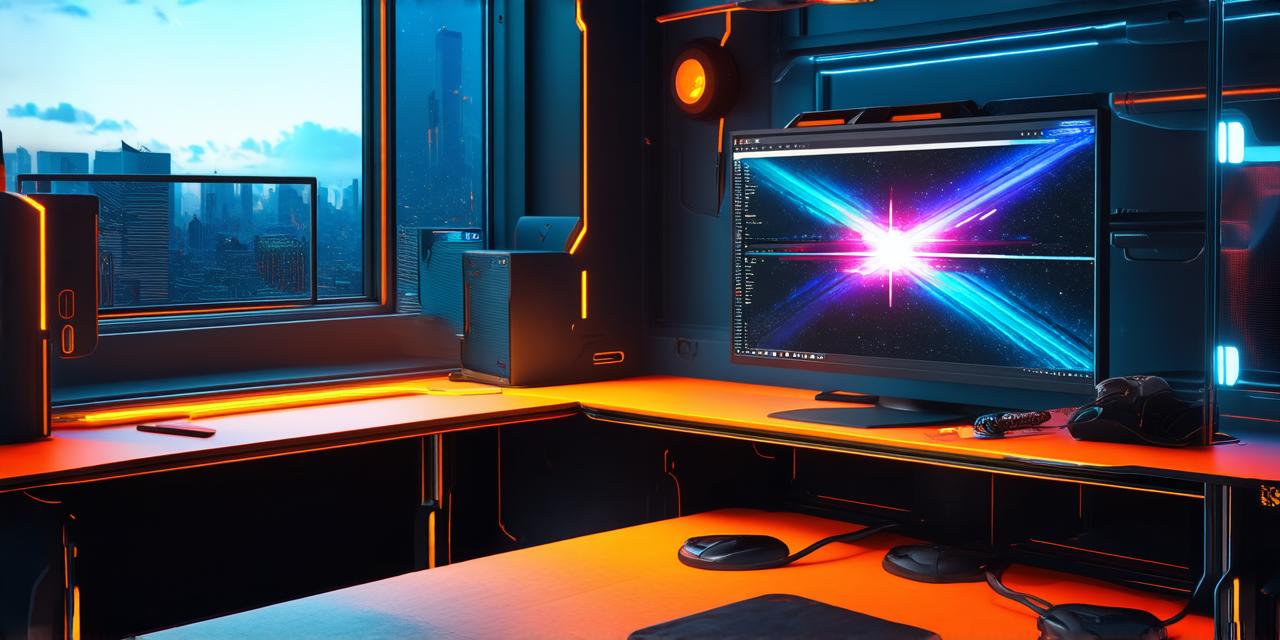
How to Create a 3D Game Using Unity: A Comprehensive Guide
Unity is a powerful and versatile game engine that allows developers to create stunning 3D games for various platforms. With its easy-to-use interface, intuitive tools, and vast community of developers, it’s no wonder why Unity has become one of the most popular game engines on the market. In this comprehensive guide, we will walk you through the process of creating a 3D game using Unity, from start to finish.
Prerequisites for Creating a 3D Game with Unity
Before diving into the world of game development with Unity, there are a few prerequisites that you should be aware of:
- A computer with sufficient hardware: To run Unity, you’ll need a computer with at least 4 GB of RAM and a dedicated graphics card.
- Basic programming skills: While Unity does come with its own scripting language (C), it’s helpful to have some basic programming knowledge before diving in.
- A passion for game development: Creating a 3D game is no easy task, and it requires a lot of creativity, patience, and dedication.
Getting Started with Unity
Once you’ve met the prerequisites, it’s time to get started with Unity. Here are the steps to follow:
- Download and install Unity: You can download the latest version of Unity from the official website (https://unity3d.com/get-unity/download). Follow the installation instructions and make sure you select the version that matches your operating system.
- Create a new project: After installing Unity, open it up and create a new 3D game project. You can choose from various templates, such as “2D Platformer”, “First Person Shooter”, or “Puzzle’.”
- Set up the scene: This involves adding game objects (such as characters, obstacles, and cameras), creating terrain, and setting up lighting.
- Add animations: Animations are an essential part of any 3D game. You can use Unity’s built-in animation tools or import animations from external software.
- Write code: Unity uses C as its scripting language, so you’ll need to learn the basics of C programming to write code for your game. There are many online resources and tutorials available to help you get started with C.
- Test and iterate: Finally, test your game regularly and make iterations based on feedback from players. This is an ongoing process, and it’s important to continuously improve your game until it’s ready for release.
Case Study: Creating a 3D Adventure Game with Unity
Step 1: Set up the Scene
The first step in creating a 3D adventure game is to set up the scene. This involves adding game objects such as characters, obstacles, and cameras, and creating terrain. In this example, we’ll create a simple dungeon-style game with a character, some walls, and a door.
Step 2: Add Animations
Animations are an essential part of any 3D game. In this example, we’ll add animations to our character so that it can walk, run, and open doors. We’ll use Unity’s built-in animation tools to create these animations.
Step 3: Write Code
Now that we have our scene set up and our animations added, it’s time to write code for our game. We’ll use C to create a simple script that allows our character to move and interact with the environment.
csharp
using UnityEngine;
public class CharacterController : MonoBehaviour
{
public float speed = 5f; // movement speed of the character
public Transform door; // reference to the door game object
void Update()
{
float horizontalInput = Input.GetAxis("Horizontal"); // get input from the player
float verticalInput = Input.GetAxis("Vertical");
Vector3 movementDirection = new Vector3(horizontalInput, 0f, verticalInput);
transform.position += movementDirection speed Time.deltaTime; // move the character
if (Input.GetButtonDown("Interact") && door != null) // check if the player presses the interact button and is near the door
{
door.localPosition = Vector3.zero; // open the door
}
}
}
Step 4: Test and Iterate
Finally, we’ll test our game regularly and make iterations based on feedback from players. In this example, we might add more obstacles, enemies, and power-ups to make the game more challenging and engaging.
Expert Opinions and Real-Life Examples
To further illustrate how to create a 3D game using Unity, let’s hear from some expert opinions and real-life examples.
Expert Opinion: “Unity is the most accessible game engine out there” – John Carmack (Founder of id Software)
John Carmack, the founder of id Software (creators of Doom and Wolfenstein), has praised Unity for its accessibility and ease of use. According to him, Unity is the most accessible game engine on the market, making it an excellent choice for beginners and experienced developers alike.
FAQs
Q: What programming languages can I use with Unity?
A: Unity supports various programming languages, including C, JavaScript, and Boo. However, C is the most popular and widely used language for game development with Unity.
Q: Can I create 2D games with Unity?
A: Yes, Unity also supports 2D game development. You can use Unity’s built-in 2D tools or import 2D assets from external software.
Q: How long does it take to create a 3D game with Unity?
A: The time it takes to create a 3D game with Unity depends on the complexity and scope of the project. Simple games can be created in a few weeks, while more complex games can take several months or even years.
Q: Do I need any previous experience to create a 3D game with Unity?
A: While having some programming and game development experience can be helpful, it’s not necessary to create a 3D game with Unity. There are many online resources and tutorials available to help beginners get started.
Conclusion
In conclusion, creating a 3D game with Unity is an accessible and exciting process. With the right tools, resources, and mindset, anyone can create engaging and immersive games using this powerful engine.