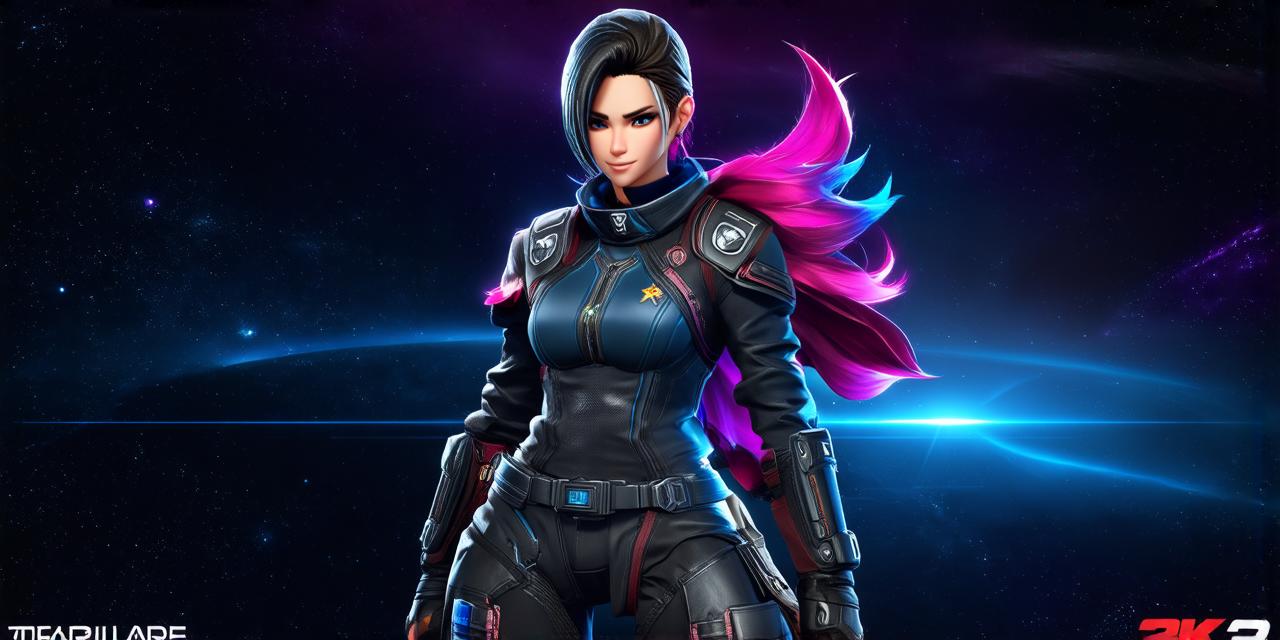
Controlling Character Movement with Keyboard Input
To control character movement with keyboard input, you can create a script that listens for keyboard inputs and updates the character’s movement accordingly. Here is an example of how to do this:
csharp
using UnityEngine;
public class PlayerController : MonoBehaviour
{
public float movementSpeed = 5f;
void Update()
{
if (Input.GetKeyDown(KeyCode.W))
{
transform.position += Vector3.forward Time.deltaTime movementSpeed;
}
if (Input.GetKeyDown(KeyCode.S))
{
transform.position += Vector3.back * Time.deltaTime * movementSpeed;
}
if (Input.GetKeyDown(KeyCode.A))
{
transform.position += Vector3.left Time.deltaTime movementSpeed;
}
if (Input.GetKeyDown(KeyCode.D))
{
transform.position += Vector3.right * Time.deltaTime * movementSpeed;
}
}
}
This script listens for the WASD keys and updates the character’s position accordingly, using the movementSpeed
variable to control how fast the character moves. You can attach this script to your character object in the Unity editor to enable keyboard movement.
Controlling Character Movement with Mouse Input
To control character movement with mouse input, you can create a script that listens for mouse inputs and updates the character’s movement accordingly. Here is an example of how to do this:
csharp
using UnityEngine;
public class PlayerController : MonoBehaviour
{
public float movementSpeed = 5f;
void Update()
{
if (Input.GetMouseButtonDown(0))
{
Vector3 lookDirection = transform.forward;
float lookAngle = Mathf.Atan2(lookDirection.y, lookDirection.x);
transform.rotation = Quaternion.Euler(new Vector3(0, lookAngle, 0));
}
if (Input.GetKeyDown(KeyCode.W))
{
transform.position += Vector3.forward * Time.deltaTime * movementSpeed;
}
}
}
This script listens for the left mouse button and updates the character’s rotation to face in the direction of the mouse cursor. It then uses the movementSpeed
variable to control how fast the character moves when the W key is pressed. You can attach this script to your character object in the Unity editor to enable mouse movement.
Controlling Character Movement with Touch Input
To control character movement with touch input, you can create a script that listens for touch inputs and updates the character’s movement accordingly. Here is an example of how to do this:
csharp
using UnityEngine;
public class PlayerController : MonoBehaviour
{
public float movementSpeed = 5f;
void Update()
{
if (Input.touchCount > 0)
{
Touch touch = Input.GetTouch(0);
if (touch.phase == TouchPhase.Began && touch.position.y < Screen.height / 2)
{
Vector3 lookDirection = transform.forward;
float lookAngle = Mathf.Atan2(lookDirection.y, lookDirection.x);
transform.rotation = Quaternion.Euler(new Vector3(0, lookAngle, 0));
}
if (touch.phase == TouchPhase.Ended && touch.position.y > Screen.height / 2)
{
if (Input.GetKeyDown(KeyCode.W))
{
transform.position += Vector3.forward * Time.deltaTime * movementSpeed;
}
}
}
}
}
This script listens for touch inputs on mobile devices and updates the character’s rotation and position accordingly. It uses the movementSpeed
variable to control how fast the character moves when the W key is pressed. You can attach this script to your character object in the Unity editor to enable touch movement.