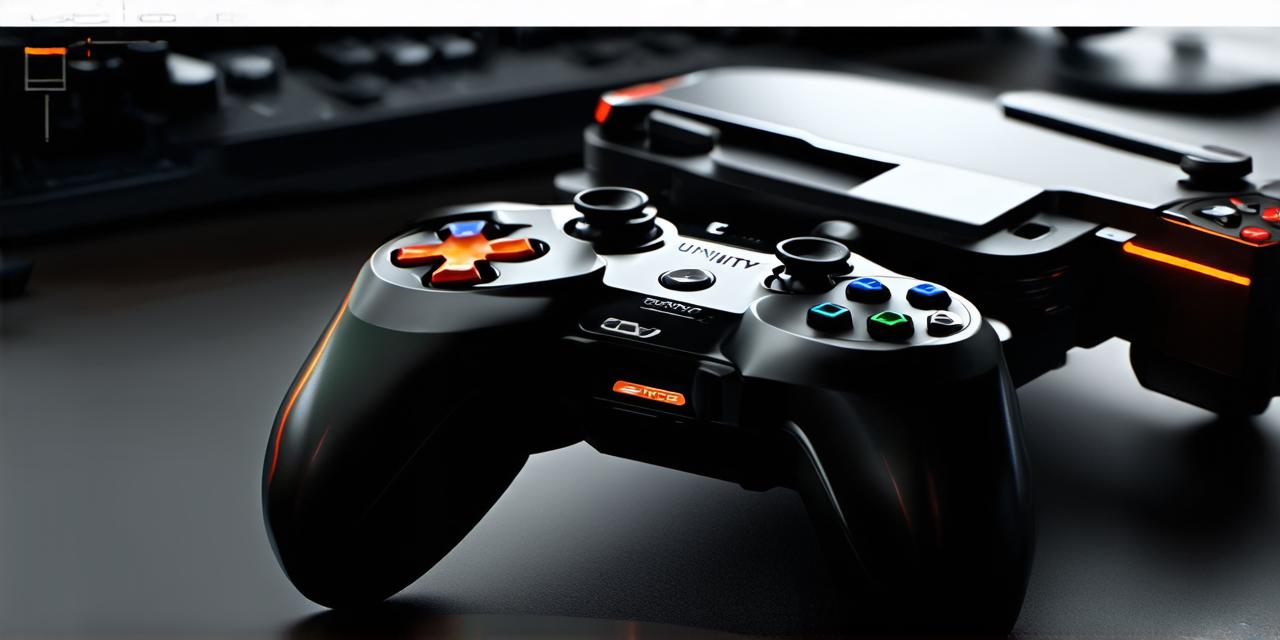
Are you looking to add some realism to your Unity projects? Then you’re in luck! In this article, we will explore how to apply gravity to objects in Unity 3D. By the end of this guide, you’ll have a solid understanding of how to create realistic physics simulations that will impress your players.
What is Gravity in Unity 3D?
Gravity is a fundamental force of nature that attracts all objects with mass towards each other. In Unity, gravity can be simulated using the rigidbody component.
This component allows you to apply forces to an object, including gravity, which will cause it to move and interact with the environment in a realistic way.
The Rigidbody Component
The rigidbody component is a powerful tool for simulating physics in Unity. It allows you to control an object’s movement, rotation, and collisions with other objects. To use the rigidbody component, simply select an object in your scene and add it to the hierarchy by right-clicking on it and selecting “Add Component” > “Physics” > “Rigidbody”.
Once the component is added, you can control its behavior using various properties and scripts. Some of the most common properties include:
- Mass: This determines how much force is needed to move the object.
- Gravity Scale: This controls how strong the gravitational force is. A higher gravity scale will cause the object to fall faster, while a lower one will make it move slower.
- Angular Velocity: This determines how fast the object spins.
- Linear Velocity: This controls how fast the object moves in all directions.
Adding Gravity to an Object
To add gravity to an object in Unity, you simply need to set the “Gravity Scale” property of the rigidbody component to a positive value. This will cause the object to fall towards the ground (or any other surface it’s on) as if pulled by gravity.
For example, let’s say we want to add gravity to a cube in our scene. We can do this by selecting the cube and adding a rigidbody component to it. Then, we can open up the component’s properties and set the “Gravity Scale” to 10. This will make the cube fall towards the ground with a force of 10 units per second squared.
Applying Forces to an Object in Unity
In addition to gravity, you can also apply other forces to objects in Unity using the rigidbody component. For example, you might want to apply a force to make an object move in a certain direction or collide with another object.
To do this, you can use the “Add Force” method of the rigidbody component. This method takes two arguments: the first is the force vector (which determines the direction and magnitude of the force), and the second is the torque vector (which controls how fast the object spins).
Here’s an example of how to apply a force to make an object move in a certain direction:
<script>
// Get the rigidbody component of the object
var rb = GetComponent<Rigidbody>();
// Calculate the force vector (in this case, we want to move the object to the right)
var force = new Vector3(10f, 0f, 0f);
// Apply the force to the object
rb.AddForce(force);
</script>
You can also use the “Add Torque” method to apply a torque to an object and make it spin faster or slower.
Collisions in Unity
In addition to gravity and forces, you can also simulate collisions between objects in Unity using the rigidbody component. This allows you to create realistic interactions between objects that will make your scenes feel more alive and immersive.
To enable collisions in Unity, you’ll need to give each object a Collider component. This component defines the shape and size of the object and allows it to interact with other colliders in the scene.
Once you have colliders on all your objects, you can use the “OnCollisionEnter” method of the rigidbody component to detect when two colliders intersect. This method takes one argument: a Collision object that represents the collision between the two objects.
Real-World Examples of Gravity in Unity 3D
Gravity is a fundamental force that has been studied extensively by scientists and engineers throughout history. In Unity, you can use this concept to create realistic simulations of movement and interaction between objects.
Whether you’re creating a planetarium simulation or a physics-based game, the rigidbody component provides a powerful tool for simulating gravity and other forces in your scenes.